Streaming Real-time Crypto Trades via WebSocket
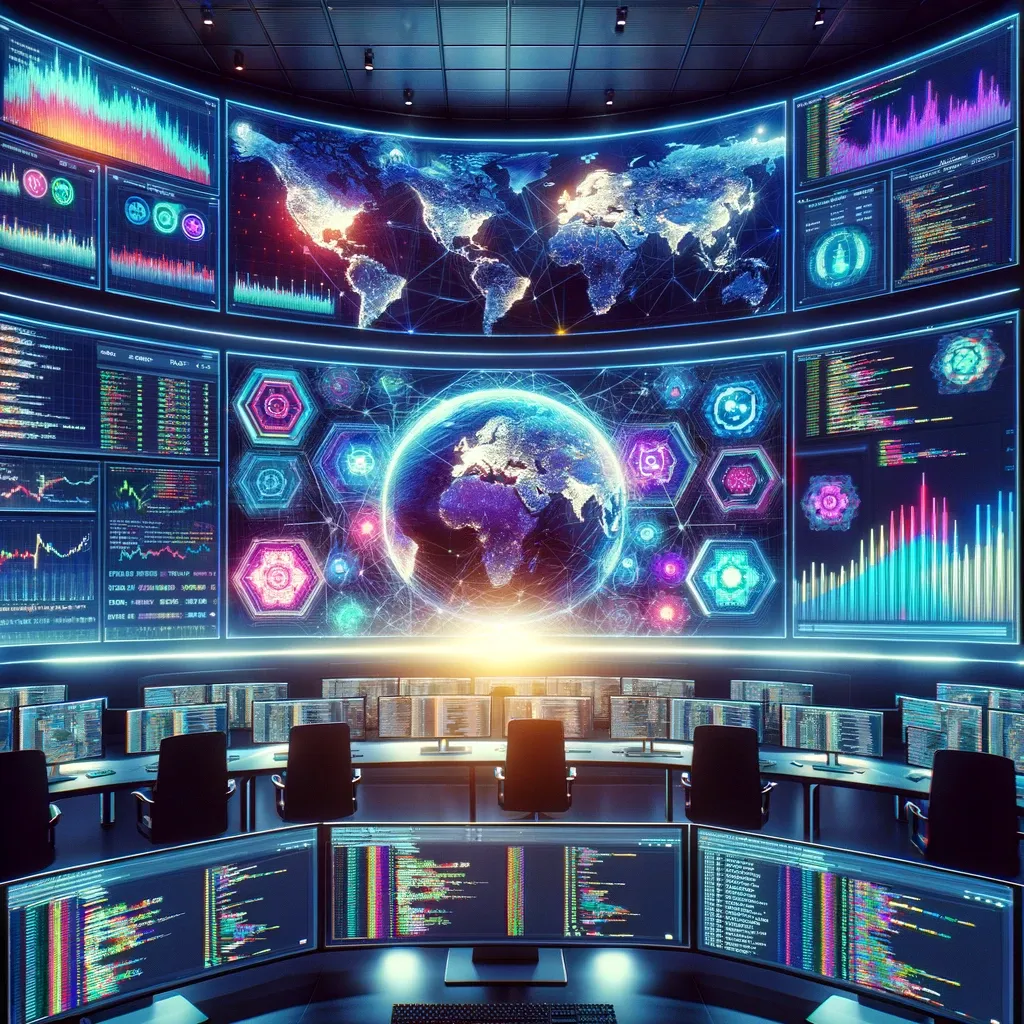
Introduction
This guide will show you how to access live cryptocurrency market data through Mobula API, utilizing WebSocket protocol in Python, JavaScript, and Java for continuous market data updates.
WebSocket Protocol
Mobula API delivers real-time information via WebSocket, a protocol allowing bidirectional communication channels over a single TCP connection, ideal for updating live data.
For accessing live data, here's the endpoint:
wss://general-api-wss-fgpupeioaa-uc.a.run.app
To start receiving specific data types, transmit a subscription message once the connection stands.
Python
In Python, employ the websocket-client
library for Mobula's WebSocket API connection.
Install the websocket-client
library with pip if it's not already installed:
pip install websocket-client
Example for fetching live trade data:
import json
import websocket
def on_message(ws, message):
print(message)
def on_open(ws):
message = {
"type": "market",
"authorization": "MOBULA-API-KEY",
"payload": {
"assets": [
{"name": "Bitcoin"},
{
"address": "0x059fd118aa8988f5e97b66fcd489765111e069c9",
"blockchain": "1",
},
],
"interval": 1,
},
}
ws.send(json.dumps(message))
if __name__ == "__main__":
ws = websocket.WebSocketApp("wss://general-api-wss-fgpupeioaa-uc.a.run.app",
on_open=on_open,
on_message=on_message)
ws.run_forever()
This Python script:
- Establishes a WebSocket connection.
- Subscribes to
BTC/WETH
for live data. - Outputs real-time messages
You can check out the documentation for more details.
JavaScript/Typescript
Before starting with JavaScript, ensure the nodejs runtime environment
is installed.
Create a package.json
including the node-fetch dependency:
{
"type": "module",
"dependencies": {
"ws": "^8.13.0"
}
}
For JavaScript, here's how you can use the WebSocket API:
import WebSocket from 'ws';
const ws = new WebSocket('wss://general-api-wss-fgpupeioaa-uc.a.run.app');
ws.onopen = () => {
const message = {
type: 'market',
authorization: 'MOBULA-API-KEY',
payload: {
assets: [
{name: 'Bitcoin'},
{
address: '0x2260fac5e5542a773aa44fbcfedf7c193bc2c599',
blockchain: '1',
},
],
interval: 1,
},
};
ws.send(JSON.stringify(message));
};
ws.onmessage = event => {
console.log(event.data);
};
This Javascript script:
- Establishes a WebSocket connection.
- Subscribes to
BTC/WETH
for live data. - Outputs real-time messages
Install necessary packages with npm install
or yarn add
. Run your script in nodejs with node your-script-name.js
or in TypeScript with ts-node your-script-name.ts
. Further details are in the documentation.
Please refer to the documentation for further details.
JavaScript in a web browser
For web implementation, craft an index.html
file, insert the code, and open it in a browser:
<!DOCTYPE html>
<html>
<head>
<title>Realtime Crypto Market Data Example</title>
</head>
<body>
<script>
const socket = new WebSocket('wss://general-api-wss-fgpupeioaa-uc.a.run.app');
ws.onopen = () => {
const message = {
type: 'market',
authorization: 'MOBULA-API-KEY',
payload: {
assets: [
{name: 'Bitcoin'},
{
address: '0x059fd118aa8988f5e97b66fcd489765111e069c9',
blockchain: '1',
},
],
interval: 1,
},
};
ws.send(JSON.stringify(message));
};
socket.onmessage = event => {
console.log(event.data);
const contentDiv = document.getElementById('content');
contentDiv.textContent += event.data;
};
socket.onerror = function (error) {
console.log(`WebSocket error: ${error}`);
};
</script>
<div id="content">
</body>
</html>
Java
For Java within a Maven project, add Java-WebSocket
and JSON
dependencies to your pom.xml:
<dependencies>
<dependency>
<groupId>org.java-websocket</groupId>
<artifactId>Java-WebSocket</artifactId>
<version>1.5.1</version>
</dependency>
<dependency>
<groupId>org.json</groupId>
<artifactId>json</artifactId>
<version>20210307</version>
</dependency>
</dependencies>
Java usage example with WebSocketClient
and JSONObject
classes:
import org.java_websocket.client.WebSocketClient;
import org.java_websocket.handshake.ServerHandshake;
import org.json.JSONObject;
import java.net.URI;
import java.net.URISyntaxException;
public class MyWebSocketClient extends WebSocketClient {
public MyWebSocketClient(URI serverUri) {
super(serverUri);
}
@Override
public void onOpen(ServerHandshake handshakedata) {
System.out.println("Opened connection");
// Construction du message à envoyer
JSONObject message = new JSONObject();
message.put("type", "market");
message.put("authorization", "MOBULA-API-KEY");
JSONObject payload = new JSONObject();
payload.put("interval", 1);
payload.put("assets", new JSONObject[] {
new JSONObject().put("name", "Bitcoin"),
new JSONObject().put("address", "0x2260fac5e5542a773aa44fbcfedf7c193bc2c599").put("blockchain", "1")
});
message.put("payload", payload);
send(message.toString());
}
@Override
public void onMessage(String message) {
System.out.println("Received: " + message);
}
@Override
public void onClose(int code, String reason, boolean remote) {
System.out.println("Closed with exit code " + code + " additional info: " + reason);
}
@Override
public void onError(Exception ex) {
System.err.println("An error occurred:" + ex.getMessage());
}
public static void main(String[] args) throws URISyntaxException {
MyWebSocketClient client = new MyWebSocketClient(new URI("wss://general-api-wss-fgpupeioaa-uc.a.run.app"));
client.connect();
}
}
This Java script:
- Establishes a WebSocket connection.
- Subscribes to
BTC/WETH
for live data. - Outputs real-time messages
Best Tips
- Address potential connection issues due to unstable internet by incorporating reconnection strategies.
- Monitor your messaging rate to adhere to Mobula API's rate limits.
- Ensure your API key's security; avoid hardcoding and opt for environment variables or secure, untracked config files.
Please refer to the documentation for further details.
Breakdown service
If you encounter any problems, please check the following points:
- Verify API key validity and permissions.
- Ensure correct WebSocket endpoint URL format.
- Confirm installation of required libraries or dependencies and language version compatibility.
Look forward to additional MobulaAPI tutorials for advanced functionalities!