How to Get Multi Wallet Crypto Historical Data via API
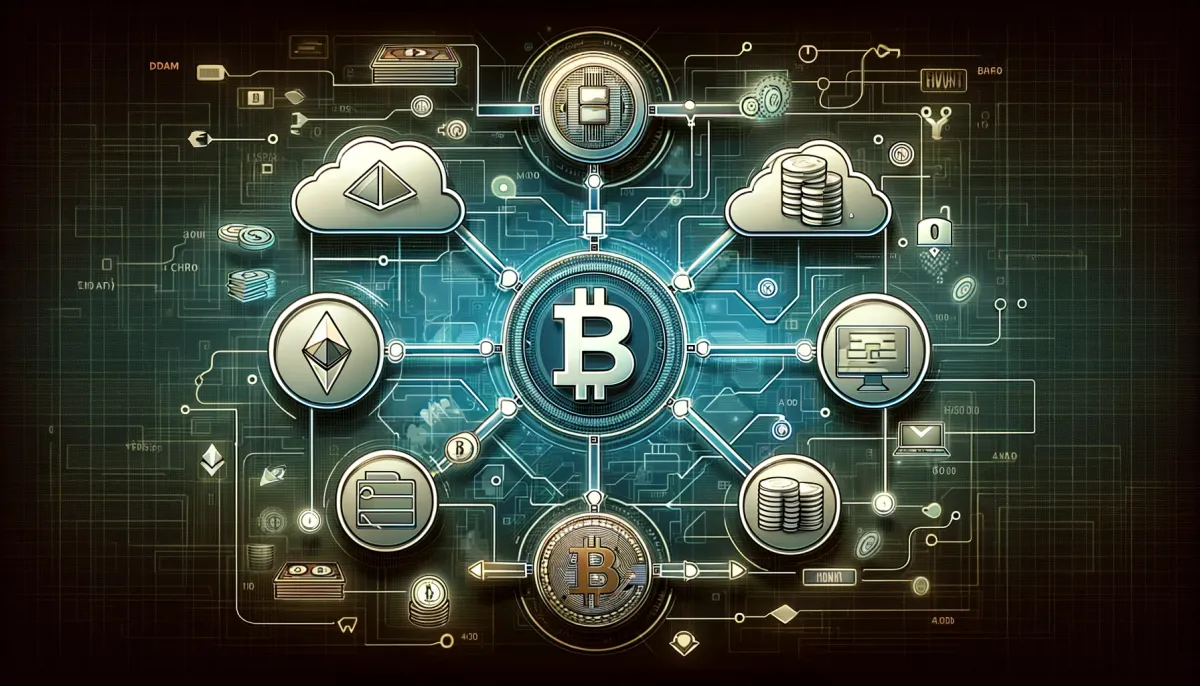
Introduction
In the dynamic and ever-evolving cryptocurrency market, developers are continually seeking efficient ways to access and leverage historical data across multi-wallet portfolios. The challenge of tracking and analyzing assets across various wallets requires a sophisticated and versatile solution. Enter Mobula API: a powerful tool designed to simplify the retrieval of multi-wallet crypto historical data for developers. This tutorial is crafted specifically for developers interested in using TypeScript, providing a step-by-step guide on how to integrate the Mobula API into your projects. By highlighting the capabilities of our platform, we aim to demonstrate how Mobula can be a pivotal tool in your development arsenal.
Prerequisites
Before embarking on this tutorial, make sure you have the following prerequisites ready:
- Mobula API Key: Access to our services is key, so sign up on the Mobula platform and obtain your API key through your user dashboard. This key is your access token to the wealth of data provided by Mobula.
Get your free API key here
- TypeScript Knowledge: A proficient understanding of TypeScript and familiarity with Node.js environments will be crucial, as the tutorial will utilize TypeScript for code examples.
- Node.js and npm Setup: Ensure your development environment includes Node.js and npm (Node Package Manager). This setup is necessary for managing project dependencies and running the TypeScript compiler.
With these prerequisites in place, you're well-equipped to leverage the Mobula API for fetching multi-wallet crypto historical data, bringing a new level of efficiency and insight to your development projects.
Setting Up Your Environment
To efficiently use the Mobula API with TypeScript, setting up a proper development environment is crucial. Follow these steps to prepare your system:
- Initialize a New TypeScript Project:
- Open your terminal or command prompt.
- Navigate to your project directory. If you don't have one, create it with
mkdir your_project_name
and thencd your_project_name
. - Initialize a new npm project by running
npm init -y
. This command creates apackage.json
file in your project directory. - Install TypeScript globally (if you haven't already) with
npm install -g typescript
. - Add TypeScript to your project as a development dependency with
npm install --save-dev typescript
. - Initialize a TypeScript configuration file,
tsconfig.json
, by runningtsc --init
. This file allows you to specify your TypeScript compiler options.
- Install Required Libraries:
- Axios: A promise-based HTTP client for making requests to the Mobula API. Install it using
npm install axios
. - Dotenv (Optional): A module that loads environment variables from a
.env
file intoprocess.env
. Useful for managing your API keys securely. Install withnpm install dotenv
.
- Axios: A promise-based HTTP client for making requests to the Mobula API. Install it using
- Set Up Your TypeScript Configuration:
- Open the
tsconfig.json
file. - Ensure the compiler options are set to your preference. For a basic setup, you might want to ensure
target
is set toes6
or higher, andmoduleResolution
is set tonode
.
- Open the
- Environment Variables:
- Create a
.env
file in the root of your project directory. - Add your Mobula API key in this file like so:
MOBULA_API_KEY=your_api_key_here
. Remember to replaceyour_api_key_here
with your actual Mobula API key.
- Create a
- Writing Your First Script:
- Create a new file named
index.ts
in your project directory. - You'll use this file to write your TypeScript code to interact with the Mobula API.
- Create a new file named
This setup prepares your development environment for fetching multi-wallet crypto historical data using the Mobula API. In the next section, we'll dive into authenticating and making requests to the Mobula API using TypeScript.
Authenticating with the Mobula API
When fetching wallet portfolio data from the Mobula API, it's essential to use the /wallet-portfolio
endpoint, which provides a comprehensive view of a wallet's holdings. To access this endpoint with TypeScript, you'll follow a similar authentication process as before, using Axios for your HTTP requests. Here's how to set up your environment and make authenticated requests to the /wallet-portfolio
endpoint.
Set Up Axios with API Key:
First, ensure your environment is set up to use Axios and dotenv (if you're handling your API key through environment variables).
- Import Axios and dotenv at the top of your
index.ts
file:
import axios from 'axios';
import dotenv from 'dotenv';
dotenv.config();
- Configure an Axios instance with your Mobula API key:
const api = axios.create({
baseURL: 'https://api.mobula.io/api',
headers: {
Authorization: `Bearer ${process.env.MOBULA_API_KEY}`
}
});
Fetching Wallet Portfolio Data:
To fetch wallet portfolio data, you'll use the /wallet-portfolio
endpoint. Here's an example function that demonstrates how to make a request to this endpoint, passing in a wallet address as a parameter:
async function fetchWalletPortfolio(walletAddress: string) {
try {
const response = await api.get(`/wallet-portfolio`, {
params: { wallet: walletAddress }
});
console.log(response.data);
} catch (error) {
console.error('Error fetching wallet portfolio:', error);
}
}
// Example usage
fetchWalletPortfolio('0xYourWalletAddressHere');
This function makes a GET request to the Mobula API, targeting the /wallet-portfolio
endpoint. It uses the wallet address you provide as a query parameter. Replace '0xYourWalletAddressHere'
with the actual wallet address you're interested in querying. The function then logs the response data or catches and logs any errors encountered during the request.
By following these steps, you can successfully authenticate and retrieve wallet portfolio data from the Mobula API using TypeScript. This approach enables you to integrate cryptocurrency portfolio information into your applications, providing valuable insights into wallet holdings.
Response
The response from the /wallet-portfolio
endpoint provides a detailed view of the wallet's portfolio, including assets held, their characteristics, and the total balance. Here's a breakdown of the response format:
- data: The primary object containing the response data.
- assets: An array of assets within the wallet. Each asset contains:
- asset: An object with detailed data about the asset, including:
- data: Nested data providing comprehensive information about each asset, such as:
- audit, blockchains, chat, etc.: Various attributes detailing the asset's properties, community links, supply data, and financial statistics.
- description: A textual description of the asset.
- id: A unique identifier for the asset.
- name, symbol: The common name and trading symbol of the asset.
- price: The current market price of the asset.
- market_cap, liquidity, volume: Financial metrics regarding the asset.
- website, twitter, discord, chat: Links to the asset's online presence and community platforms.
- data: Nested data providing comprehensive information about each asset, such as:
- cross_chain_balances: An object representing the balances of the asset across different blockchains (if applicable).
- estimated_balance: The estimated balance of the asset within the wallet, possibly aggregating across multiple blockchains.
- price: The current price of the asset.
- token_balance: The specific balance of the token within the wallet.
- asset: An object with detailed data about the asset, including:
- total_wallet_balance: A numeric value representing the total estimated balance of the wallet in a standard currency (e.g., USD).
- wallet: The wallet address as a string.
- assets: An array of assets within the wallet. Each asset contains:
- lastUpdated: An object that may contain timestamps or other data indicating when the information was last updated.
This structured response allows developers to extract and analyze a wide range of data about a wallet's holdings, from basic financial statistics to detailed asset descriptions and community engagement links. With this information, developers can create comprehensive portfolio analysis tools, dashboards, and other applications that provide valuable insights to users about their cryptocurrency investments.
Practical Applications
The detailed data provided by the Mobula API, especially from the /wallet-portfolio
endpoint, can be leveraged in various practical applications to enhance cryptocurrency portfolio management and analysis. Here are some ideas on how to use this data effectively:
Portfolio Tracking
- Automated Portfolio Overview: Develop an application that automatically updates and displays a comprehensive overview of a user’s cryptocurrency portfolio. This can include current asset values, total portfolio balance, and asset distribution. The real-time nature of the API allows for up-to-the-minute accuracy in portfolio valuation.
- Alerts and Notifications: Implement a system that notifies users of significant changes in their portfolio's value or the value of individual assets. By tracking historical data, you can identify trends and set alerts for specific thresholds, ensuring users are informed of important market movements.
Performance Analysis
- Historical Performance Tracking: Create tools that analyze the historical performance of a user's portfolio. By comparing current asset values to historical data, users can see how their investments have grown or declined over time, offering insights into their investment strategies' effectiveness.
- Asset Correlation Analysis: Utilize historical data to examine the correlation between different assets in a portfolio. This analysis can help users understand diversification in their portfolio and identify assets that move in tandem or inversely, aiding in risk management.
Investment Strategy Optimization
- Trend Identification and Predictive Analytics: Apply machine learning algorithms to historical data to identify market trends and predict future asset performance. This can guide users in making informed investment decisions, potentially increasing their returns.
- Risk Assessment and Management: Analyze historical price volatility and liquidity of assets to assess portfolio risk. This information can help users adjust their investment strategies, balancing potential returns against their risk tolerance.
Custom Reporting
- Personalized Financial Reports: Generate customized reports for users, highlighting key metrics such as portfolio growth, asset distribution, and performance against market benchmarks. These reports can be tailored to individual preferences, providing valuable insights in a user-friendly format.
- Tax Reporting and Optimization: Use historical transaction data to create detailed reports for tax purposes, helping users track capital gains and losses. This can also include recommendations for tax-loss harvesting opportunities, optimizing their tax situation.
Educational Tools
- Market Analysis and Education: Build educational platforms that use historical data to teach users about market analysis, investment strategies, and cryptocurrency fundamentals. This can include interactive charts, performance simulations, and scenario analysis tools.
By leveraging the comprehensive data available from the Mobula API, developers can create a wide range of applications and tools that not only enhance the user experience for cryptocurrency investors but also provide deep insights and analytics to inform their investment decisions. These practical applications demonstrate the versatility and value of integrating historical data into cryptocurrency portfolio management and analysis tools.
Conclusion
Leveraging the Mobula API to access and analyze multi-wallet crypto historical data opens up a plethora of opportunities for developers to create sophisticated tools and applications that significantly enhance the cryptocurrency investment experience. From real-time portfolio tracking to in-depth performance analysis and strategy optimization, the practical applications of this data are vast and varied. By integrating these capabilities, developers can offer users insightful, data-driven perspectives on their investments, empowering them to make more informed decisions and potentially achieve better financial outcomes.
Additional Resources
To further explore the capabilities of the Mobula API and to enhance your development skills in the realm of cryptocurrency data analysis, consider the following resources:
- Mobula API Documentation: Dive deeper into the specifics of the API endpoints, data formats, and authentication mechanisms by visiting Mobula's API documentation.
- TypeScript Documentation: For developers utilizing TypeScript to interact with the Mobula API, the official TypeScript documentation offers a comprehensive guide to the language and its features.
- 24/7 Support on Telegram: For around-the-clock support and queries related to the Mobula API, connect with our Create a Room with Mobula. This resource is invaluable for getting immediate assistance and answers to your development questions.
By leveraging these resources and continually exploring the dynamic field of cryptocurrency data analysis, developers can expand their skill set, enhance their applications, and contribute to the growing ecosystem of financial technology solutions.