How to Create a Web3 Wallet with API
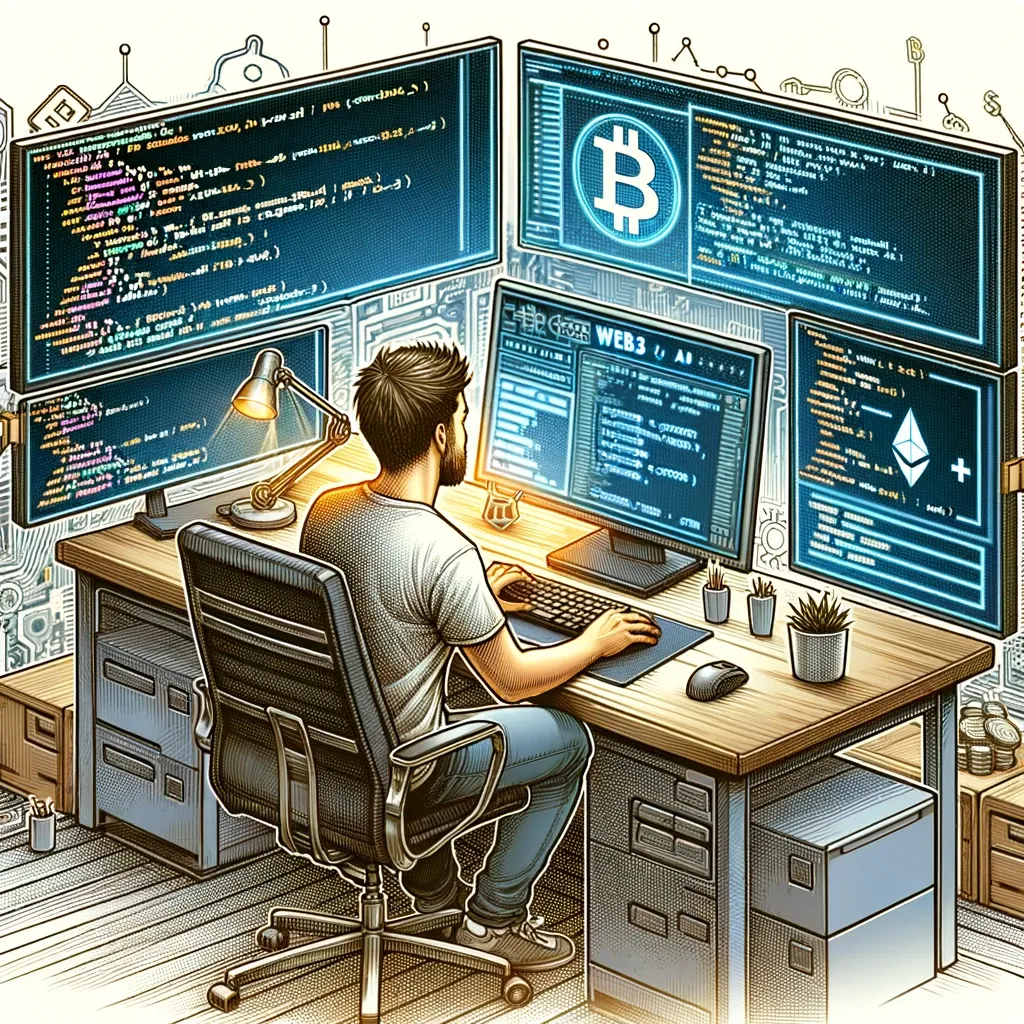
In the fast-changing world of Web3 and blockchain, developers are creating a new generation of user-focused Web3 wallets. Driven by the need for better interfaces and broader applications, they're using sophisticated tools to develop advanced wallets. Let's explore Mobula's crypto Wallet API and its potential for building cutting-edge wallets.
Top Crypto Wallet API for Developing Advanced Web3 Wallets with Mobula
Developing decentralized applications (dapps) and Web3 wallets requires easy access to both current and past crypto address data. However, gathering this data can be difficult without the right technology.
This is where Mobula's leading crypto Wallet API becomes crucial, streamlining the data access process.
A few lines of code allow access to a wealth of historical data for any crypto address with Mobula's Wallet API. This includes native transactions, ERC-20 transfers, or token balances, providing developers with essential insights. With a straightforward three-step process, one can retrieve historical crypto data!
The initial step is acquiring a Mobula Web3 API key on your dashboard:
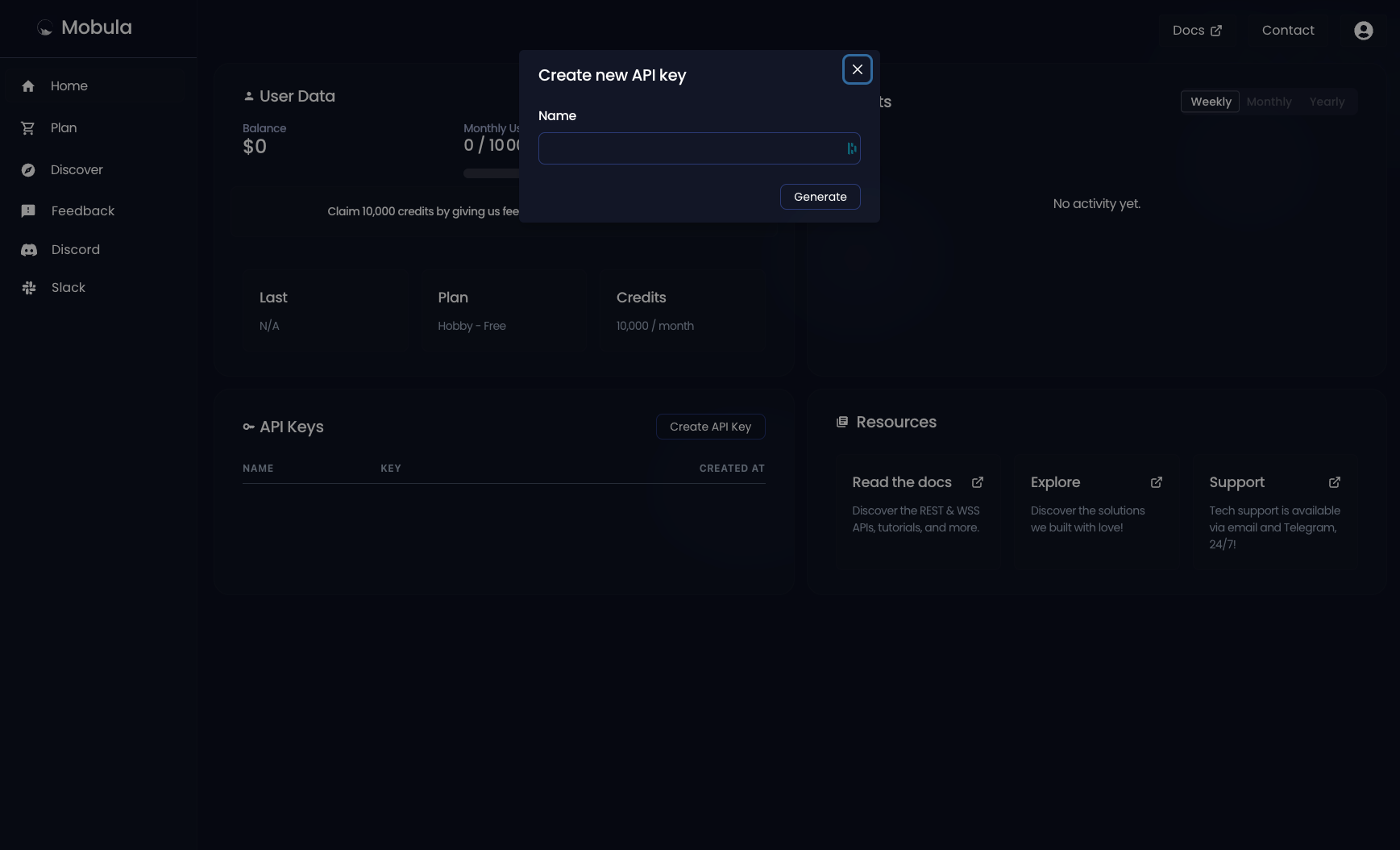
The next step includes several smaller actions. First, install the Mobula SDK:
npm install mobula-sdk
Next, add the following to your index.js file:
import { Mobula } from "mobula-sdk";
const mobula = new Mobula("YOUR_API_KEY_HERE");
mobula
.fetchWalletTransactions({
wallet: "0x77A89C51f106D6cD547542a3A83FE73cB4459135",
})
.then((response) => {
console.log(response);
})
.catch((error) => {
console.error("Error:", error);
});
You'll need to adjust a few things at this point. To do this, ensure you consult Mobula's comprehensive documentation on the crypto wallet history API. After adjustments, run your code!
Mobula's Wallet API isn't just for historical data; it enables real-time data access, allowing the creation of live wallet trackers. For guidance on setting up a wallet tracker with alerts, visit Mobula's blog for articles on developing an on-chain wallet tracker with Web3 alerts.
Remember, a wallet API is just one of the tools needed for building advanced wallets or dapps. Explore Mobula's full range of blockchain development tools. Mobula's blog also offers valuable tutorials and guides, such as using the best block explorer API for accessing blockchain data, tutorial to setup websockets and more.
Wallet Bundle
The Wallet Bundle feature in our crypto API offers a powerful solution for querying multiple wallets simultaneously, streamlining the process of aggregating and analyzing data across different blockchain addresses. This functionality not only enhances efficiency but also provides a comprehensive view of assets distributed across various wallets.
By utilizing the Wallet Bundle, developers can implement a more cohesive and unified approach to managing and displaying crypto assets, ensuring users gain a holistic insight into their investments. Whether you're developing a portfolio management tool, a financial tracking application, or simply looking to offer a more integrated user experience, the Wallet Bundle is your gateway to simplified and centralized data access.
Example:
const options = {method: 'GET'};
fetch('https://api.mobula.io/api/1/wallet/portfolio?wallets=0x99fB00369e6a5C1963dCd32Eba99D7B21c5a37D8%2C0xaF88370abD82EC6943cdB3D4ec7b764B92c35B43', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
Response format
{
"data": {
"total_wallet_balance": 3097.63376096095,
"wallets": [
"0x99fb00369e6a5c1963dcd32eba99d7b21c5a37d8",
"0xaf88370abd82ec6943cdb3d4ec7b764b92c35b43"
],
"total_realized_pnl": 1719.7395,
"total_unrealized_pnl": 809.8978,
"assets": [
{
"asset": {
"name": "StaySAFU",
"symbol": "SAFU",
"id": "3",
"contracts": [
"0x890cc7d14948478c98a6cd7f511e1f7f7f99f397"
],
"logo": "https://metacore.mobula.io/4ed4daf3f124fae650cfab146ae7348cdb62920bc25bf6408698654835192a1b.png"
},
"realized_pnl": -1.2758,
"unrealized_pnl": -6.2797,
"allocation": 0.01,
"price": 2.76659112182243,
"price_bought": 41.36223650624161,
"total_invested": 473.241,
"min_buy_price": 18.252545015879853,
"max_buy_price": 57.65213010837925,
"estimated_balance": 0.31653615626191806,
"token_balance": 0.114413783,
"cross_chain_balances": {
"BNB Smart Chain (BEP20)": {
"balance": 0.11441378299999999,
"balanceRaw": "114413783",
"chainId": "56",
"address": "0x890cc7d14948478c98a6cd7f511e1f7f7f99f397"
}
},
"contracts_balances": [
{
"balance": 0.11441378299999999,
"balanceRaw": "114413783",
"chainId": "56",
"address": "0x890cc7d14948478c98a6cd7f511e1f7f7f99f397",
"decimals": 9
}
]
},
{
"asset": {
"name": "Mobula",
"symbol": "MOBL",
"id": "222",
"contracts": [
"0x33b3a50c766dd3e61b1e90b251390e7c28aefb7c",
"0xae85d5aa526b1ecc5e90e466cbd2bdec22c606ff",
"0x5fef39b578deeefa4485a7e5944c7691677d5dd4"
],
"logo": "https://metacore.mobula.io/16aef356ede457a7baf3c0e7ddc49dc45da3f0d560d39832985502162f6c89f5.png"
},
"realized_pnl": 0,
"unrealized_pnl": 0,
"allocation": 0,
"price": 0,
"price_bought": 0,
"total_invested": 0,
"min_buy_price": 0,
"max_buy_price": 0,
"estimated_balance": 0,
"token_balance": 1023,
"cross_chain_balances": {
"Polygon": {
"balance": 1023.0000000000001,
"balanceRaw": "1023000000000000131072",
"chainId": "137",
"address": "0x5fef39b578deeefa4485a7e5944c7691677d5dd4"
}
},
"contracts_balances": [
{
"balance": 1023.0000000000001,
"balanceRaw": "1023000000000000131072",
"chainId": "137",
"address": "0x5fef39b578deeefa4485a7e5944c7691677d5dd4",
"decimals": 18
}
]
},
{
"asset": {
"name": "BNB",
"symbol": "BNB",
"id": "100001566",
"contracts": [
"0xbb4cdb9cbd36b01bd1cbaebf2de08d9173bc095c",
"0xdff772186ace9b5513fb46d7b05b36efa0a4a20d",
"0xb8c77482e45f1f44de1745f52c74426c631bdd52"
],
"logo": "https://metacore.mobula.io/4222eebc08140e722f50099fc8322b28e7c52e1abb92897e716cb2dc89598634.png"
},
"realized_pnl": -209.1623,
"unrealized_pnl": 91.6837,
"allocation": 3.46,
"price": 581.6063770506221,
"price_bought": 440.38727042489046,
"total_invested": 9430.25358954975,
"min_buy_price": 242.52631578947367,
"max_buy_price": 652.2424242424242,
"estimated_balance": 107.03133636414849,
"token_balance": 0.1840271025,
"cross_chain_balances": {
"BNB Smart Chain (BEP20)": {
"balance": 0.18402710256763155,
"balanceRaw": "184027102567631552",
"chainId": "56",
"address": "0xbb4cdb9cbd36b01bd1cbaebf2de08d9173bc095c"
}
},
"contracts_balances": [
{
"balance": 0.18402710256763155,
"balanceRaw": "184027102567631552",
"chainId": "56",
"address": "0xeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee",
"decimals": 18
}
]
},
{
"asset": {
"name": "BUSD",
"symbol": "BUSD",
"id": "100001586",
"contracts": [
"0x4fabb145d64652a948d72533023f6e7a623c7c53",
"0xe9e7cea3dedca5984780bafc599bd69add087d56",
"0x19860ccb0a68fd4213ab9d8266f7bbf05a8dde98",
"0x5d9ab5522c64e1f6ef5e3627eccc093f56167818",
"0x6ab6d61428fde76768d7b45d8bfeec19c6ef91a8",
"0x7b37d0787a3424a0810e02b24743a45ebd5530b2",
"0xdd96b45877d0e8361a4ddb732da741e97f3191ff",
"0x4bf769b05e832fcdc9053fffbc78ca889acb5e1e"
],
"logo": "https://metacore.mobula.io/56e93eb2eb1f082fab53de6f20642c21467032ce91101d5db299b48f216059d5.png"
},
"realized_pnl": 2.6554,
"unrealized_pnl": 0,
"allocation": 0,
"price": 0.9999199481365911,
"price_bought": 1.0004422945454985,
"total_invested": 1543.0906290137955,
"min_buy_price": 0.9941223364017048,
"max_buy_price": 1.0395851915163234,
"estimated_balance": 0,
"token_balance": 0,
"cross_chain_balances": {},
"contracts_balances": [
{
"balance": 0,
"balanceRaw": "0",
"chainId": "56",
"address": "0xe9e7cea3dedca5984780bafc599bd69add087d56",
"decimals": 18
},
{
"balance": 0,
"balanceRaw": "0",
"chainId": "1",
"address": "0x4fabb145d64652a948d72533023f6e7a623c7c53",
"decimals": 18
}
]
},
{
"asset": {
"name": "CryptoAI",
"symbol": "CAI",
"id": "101679199",
"contracts": [
"0xf36c5f04127f7470834ed6f98bddc1be62aba48d"
],
"logo": "https://metacore.mobula.io/38cca4128883ec20d57420114db385fc40de493b876c9df21d4a97824012cbaf.png"
},
"realized_pnl": 1230.186,
"unrealized_pnl": -2.1194,
"allocation": 0.02,
"price": 0.008915407248101831,
"price_bought": 0.056465844807023984,
"total_invested": 7004.986,
"min_buy_price": 0.04606675043007739,
"max_buy_price": 0.056470629032258066,
"estimated_balance": 0.5086030603254337,
"token_balance": 57.0476531438,
"cross_chain_balances": {
"Ethereum": {
"balance": 57.04765314386395,
"balanceRaw": "57047653143863943168",
"chainId": "1",
"address": "0xf36c5f04127f7470834ed6f98bddc1be62aba48d"
}
},
"contracts_balances": [
{
"balance": 57.04765314386395,
"balanceRaw": "57047653143863943168",
"chainId": "1",
"address": "0xf36c5f04127f7470834ed6f98bddc1be62aba48d",
"decimals": 18
}
]
},
]
}
}
What You Can Create with the Leading Web3 API
Mobula's API, exemplifies the potential of utilizing Mobula's Blockchain API, Wallet API, Streams API, and more. This on-chain, real-time data tool crafted by Mobula's developers showcases the capabilities for developers and entrepreneurs alike. Mobula API has supported numerous traders in devising crypto trading tactics and investors in seizing investment opportunities. Amidst the cryptocurrency market's exciting price surges, Mobula API offers in-depth market insights.
For traders and investors searching for the next big coin, Mobula API is a valuable resource. The blog also offers guidance on token analysis before investment, such as in the "How to get real-time Crypto Data With Websockets", detailing important metrics and statistics for speculative predictions.