Get Crypto Data with Mobula API and Typescript
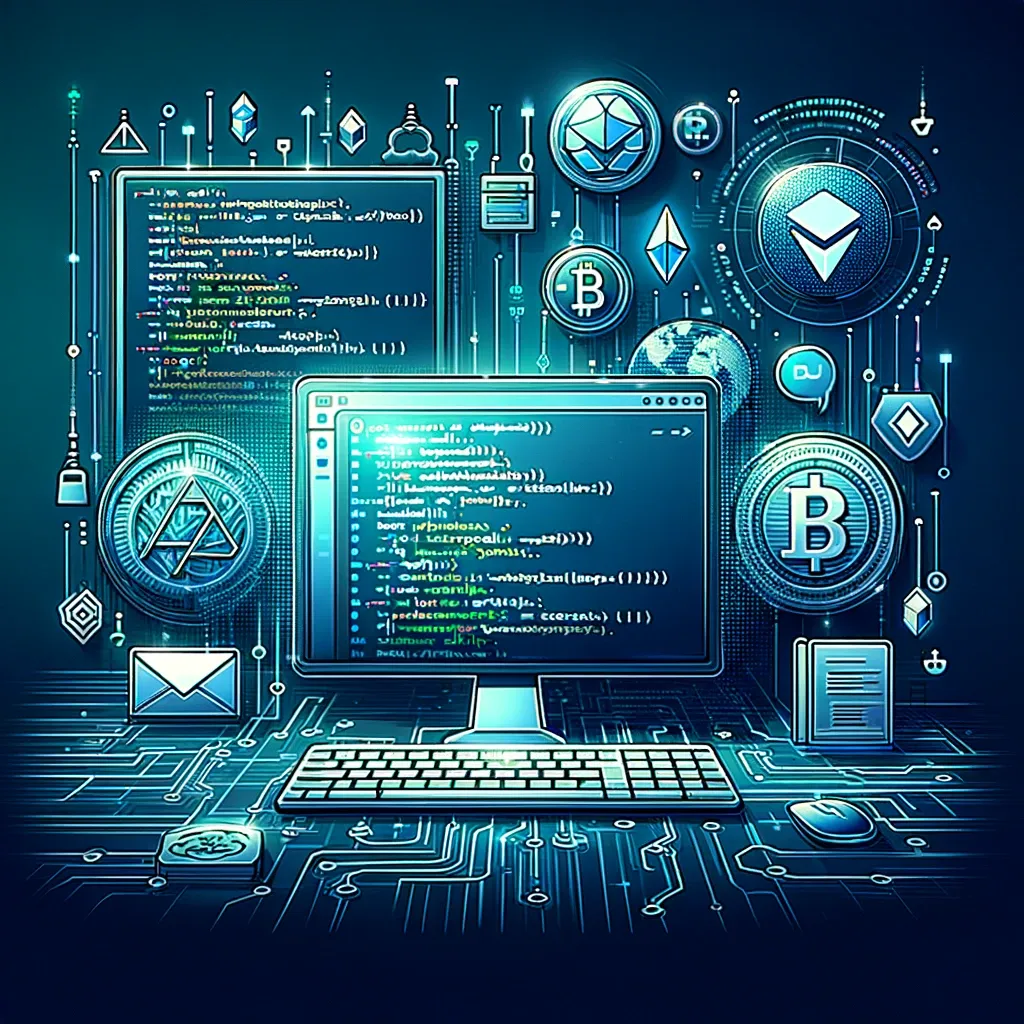
Introduction:
This guide provides a step-by-step approach to efficiently retrieve cryptocurrency data using the Mobula API and Typescript.
Mobula provides curated datasets for builders: market data with Octopus, wallets data, metadata with Metacore, alongside with REST, GraphSQL & SQL interfaces to query them.
Prerequisites:
- Node.js and npm: Ensure you have Node.js (version 14 or later) and npm installed on your system. You can download them from https://nodejs.org/.
- Mobula API Key: Obtain a free API key by following the instructions at https://docs.mobula.io/api-reference/authentication.
- Basic TypeScript Knowledge: Familiarity with TypeScript syntax, data structures, and libraries will be helpful.
Setting Up the Project:
Create a new directory for your project and navigate to it in your terminal:
mkdir crypto-data-extractor
cd crypto-data-extractor
Initialize npm Project:
npm init -y
Install Dependencies:
Install the required dependencies using npm:
npm install mobula-sdk @types/mobula-sdk
Writing the TypeScript Code:
- Create a TypeScript File:
Create a new TypeScript file (e.g., index.ts) in your project directory.
- Import Libraries and Set API Key:
Import the mobula-sdk library and set your Mobula API key:
import { Mobula } from "mobula-sdk";
// Set your Mobula API key
const mobula = new Mobula("YOUR_API_KEY_HERE");
Replace YOUR_API_KEY with your actual API key.
- Define Assets and Exchanges:
Create a dictionary to specify the assets you want to fetch data from
const assetsDict: string[] = {
['Bitcoin', 'Pepe', 'BNB']
};
- Fetch Market Data:
Iterate over the assetsDict and use the fetchAssetMarketData() function to retrieve market data for each asset:
import { Mobula } from "mobula-sdk";
const mobula = new Mobula("YOUR_API_KEY_HERE");
const assetsDict: string[] = ['Bitcoin', 'Pepe', 'BNB'];
async function main() {
for (const asset of assetsDict) {
try {
const response = await mobula.fetchAssetMarketData({ asset });
console.log(response); // Process and store the data as needed
} catch (error) {
console.error(`Error fetching data for ${asset}:`, error);
}
}
}
main();
This code snippet demonstrates how to fetch market data, extract relevant information, and prepare it for storage. You can choose to store the data in a database, CSV file, or any other suitable format based on your needs.
Storing the Data (Optional):
If you want to store the retrieved data for further analysis or historical reference, you can use a database like Supabase, SQLite or MongoDB. The specific implementation will depend on your chosen database and its integration with TypeScript.
Running the Code:
- Compile TypeScript:
Use the TypeScript compiler (tsc) to compile your TypeScript code to JavaScript:
tsc index.ts
- Run the JavaScript Code:
Run the generated JavaScript file (e.g., index.js) using Node.js:
node index.js
This will execute your code and fetch the crypto data from the Mobula API. You can then process and store the data as needed.
Additional Considerations:
- Error Handling: Implement robust error handling mechanisms to gracefully handle API errors and ensure your code's resilience.
- Rate Limiting: Adhere to Mobula's rate limits to avoid being throttled or blocked. Consider using exponential backoff or other strategies to manage API requests effectively.
- Data Cleaning and Preprocessing: Clean and preprocess the retrieved data to ensure its accuracy and consistency before analysis.
- Data Storage and Management: Choose appropriate data storage solutions (e.g., databases, CSV files) based on your data volume and access patterns.
- Security: Implement security measures to protect your API keys and sensitive data.
I hope this comprehensive guide helps you effectively extract crypto data using the Mobula API and TypeScript!